Navigate Through This Post:
Final project for Flatiron School
For my final project with Flatiron School’s Software Engineering Program, I decided that I wanted to address a gap in browser-based community games. Ludonarrative dissonance, the uncomfortable disjoint between game play and game story, is rife in pet sites because the interactions tend to be simple and the technical implementation tends to be an afterthought compared to cosmetic items. This leads to elaborate dress-up features, but tensions with keeping your pets fed because acquiring food is challenging, in one of my favorite pet sites. The problems also occur when breeding is a part of the game – the site can rapidly generate too many pets, and people get emotionally attached to their pets, or want to participate in an animal husbandry economy.
I wanted to make a game that addresses this gap by making navigating through the site be an engaging, fresh experience every day by pulling from the highly replayable Rogue and its genre-defining procedurally generated environments. If acquiring items happens as part of regular play activities that make sense, then people will feel engaged and rewarded instead of like they are grinding for items. Additionally, these browser games can be time sinks on low-reward activities. I would like to reward people for spending that time on healthy activities, by granting rewards to people who synchronize their health tracker data to the petsite and have completed healthy activities. Someone without a lot of time to spend grinding would then not have to choose between taking a walk or playing the game.
Another issue I have with pet collecting games in general, and this extends beyond the petsite genre into games like the Pokemon franchise, is that the pets themselves lose value as unique entities with their own motivations the more of them that you have. Games that incentivize amassing a large collection of, narrative-wise, living breathing animals don’t want players to think about the unfortunate implications that animal hoarding on that scale create. And in practice, most players interact only with their favorite few anyway. I would like to make a game that prevents this issue by focusing on high-quality interactions with pets – funny incidents while out walking, the adventures that a player goes on with their pet, dress up, affection and sharing food. The gameplay loop of most petsites is to press a button to automatically feed. This is not as satisfying, but I knew going into this project that pressing a button to automatically feed is at least a starting point.
Finally, I wanted to make the highly visually oriented world of petsites from the ground up to be an engaging experience for screen reader users. I am not a screen reader user myself. When I worked for a medical record company, I felt that it deeply unfair that it appeared one of my co workers was increasingly sidelined for decreasing vision. He could only help support a legacy product that was screen reader compatible – the modern GUI product didn’t look like it worked with his accessibility tools. I don’t know the whole story, but the way that played out looked bad for the rest of the staff. It’s stuck with me how hostile computers can be for accessibility purposes, and for that reason I want to release a game that is 100% compatible with screen reading technology.
While I have not achieved this goal wholly at this time, I have created a pre-alpha that demonstrates where I would like to take this concept.
Project Requirements from Flatiron
The listed requirements below are guidelines that should help you to determine what the complexity of your project should be. They are not hard and fast rules, and final project approval is up to your leads and SECs, who will be acting as project managers.
Backend
Your project must use a non-trivial Rails backend. Consult the following list for examples of things to include. You do not need to include all of these things, and the final decision of what must be included will be up to your project managers.
Auth
Tests
Multiple has_many_through relationships
Seeds from a complex data set
Custom routes
Custom controller/model methods
Basic database query optimizations
Background jobs for slow actions
Sockets or email integration
One significant refactor
Validation
Frontend
Your product must use a React based frontend. Consult the following list for examples of things to include. You do not need to include all of these things, and the final decision of what must be included will be up to your project manager (your instructor).
Auth
Tests
Interacting with a complex API
Redux
Custom CSS
One significant refactor
Project Vision
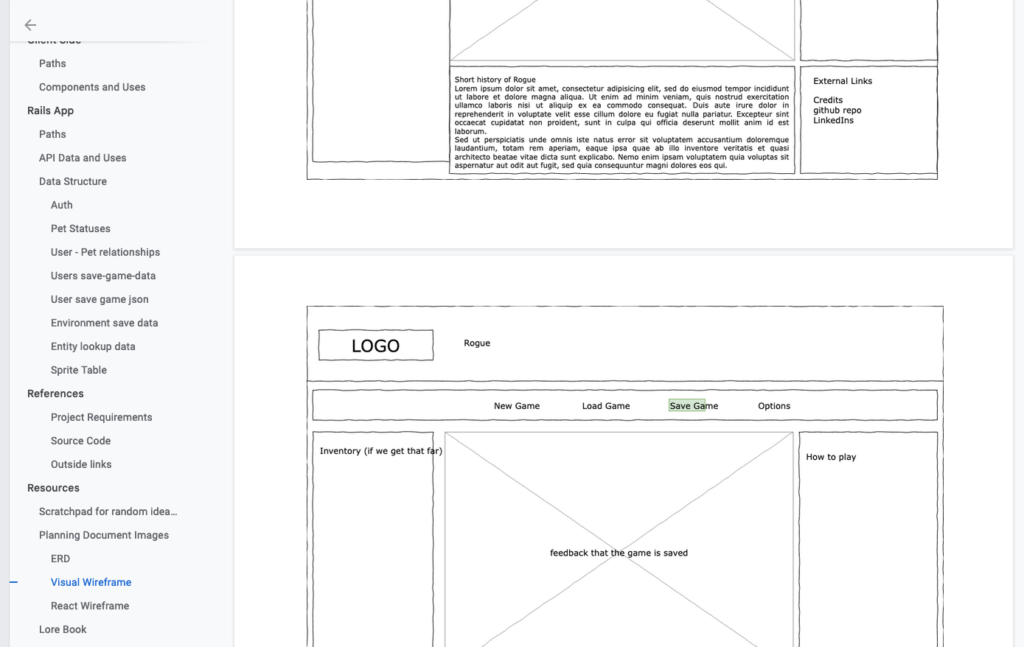
Start with what you want, then apply reality
For this project, I had the following vision story, which I later cut down to an achievable scope for the Flatiron Phase 5 final project.
Project Story
The Guild of the Magi is a petsite that allows a user to focus on building a deep, satisfying relationship with their virtual pets.
Collect-them-all gameplay is satisfied by allowing players to build relationships with their friends' pets, so they do not have to maintain a large quantity of hoarded animals.
Players' pets may gift items to other players. Pets may learn who to gift/trade with because the player character takes the party adventuring. This aspect of the game is a roguelike MUD (bones file style).
Pets may also learn who to gift/trade with because a player who visits another player's profile and interacts with the pets will weight that account for trading.
Pets may acquire more items / be more inclined to gift and trade the more time or distance a player traverses in the MUD.
Alternatively, as a stretch goal, if a player's health activity is high that day a player can get dungeon crawling credit in the form of similar knock-on effects as a single dungeon crawl per 1/2 hour of cardio or 4000 steps.
Wild pets may join a player's party if they are welcomed enough when wild pets come to visit.
Players may also breed pets, modify the habitat, and feed the pets to change their appearance and abilities.
Pets have a personality and a mood, which will affect how they respond to caretaking tasks.
Reality Ensues
The actual scope of this project was obviously much reduced as I had about ten days to turn in what I was going to produce.
Core user Stories
When I load the website, I can make an account or log in
https://app.shortcut.com/mackenzie-harrison/story/1565
https://app.shortcut.com/mackenzie-harrison/story/1568
After I am logged in, I can log out
https://app.shortcut.com/mackenzie-harrison/story/1567
The pet’s needs decrease over time
https://app.shortcut.com/mackenzie-harrison/story/1667
When pet is hungry, I can feed it
https://app.shortcut.com/mackenzie-harrison/story/1572
When pet is bored, I can play with it
https://app.shortcut.com/mackenzie-harrison/story/1574
https://app.shortcut.com/mackenzie-harrison/story/1577
After enough time, pet grows into an adult character
Owner/guest relationship to pet controls what actions are available
https://app.shortcut.com/mackenzie-harrison/story/1669
False Starts
Converting Logic from JavaScript To TypeScript
In Phase 3, Seo Choi and I made a very simple HTML5 game board with the intent of implementing different Roguelike genre features later. Seo loves the endless varieties of Roguelike combat that have sprung up over the years, and I love the procedurally generated exploration. In this phase, we built a board that would be able to provide some game entities ( a player, an enemy that would move when the player moved, and an end condition entity that when the player reached it would end the game. ) That was quite the challenge by itself. I did Phase 4 as a prototype of the pet vital statistics and degradation mechanics in TypeScript in the first place. TypeScript wasn’t the hardest to learn, but a challenging aspect for me was event typing. In C++, which is the strongly typed language I’m used to but never got far in, there was a fairly 1:1 map between data structures and data types. While I’m sure custom typing and structuring is part of more advanced C++ projects, and I may have even done some of these, it’s also been nearly ten years. So it took me a bit to figure out React state typing, creating custom types, and even things like keyboard events have a type.
const handleKeyPress = useCallback((event: KeyboardEvent) => {
handleKey(event);
}, []);
consthandleKey = (e: KeyboardEvent) => {
switch (e.keyCode) {
case38:
case87:
//upsetPetPosition((current) => ({
x: current.x,
y: current.y - 16
}))
break;
//......
}
}
Handling multiple tables in the same API call
This issue has its own extended writeup here. One of the constraints that I placed on this project is, to support the issue I have with petsite animal hoarding, that pets can have relationships with multiple players. That meant that in order to display the data in the vital statistics table, the API that I created needed to select against multiple tables and serialize the data appropriately for the frontend. This stumped multiple people and took a few days to work out, but I’m glad that I did it this way because sticking to my values on this issue also helped me learn how to handle more complex requests.
Changing the backend to avoid premature optimization
I spent too much time on the Postgres database solving problems that I probably won’t have for years if I stay with this project. Getting stressed about read performance was an ineffective use of my time.
It was, however, a good idea to start with a more flexible database structure that allows me to make changes to my frontend and middleware by having fields that store JSONs instead of needing to drop and recreate tables constantly.
Before | After |
![]() | ![]() |
Achieved Progress
The reduced scope I aimed for was to be able to show a login cycle, some pet interactions, and movement in a game board area. I achieved that, and I am looking forward to getting closer to my initial vision.
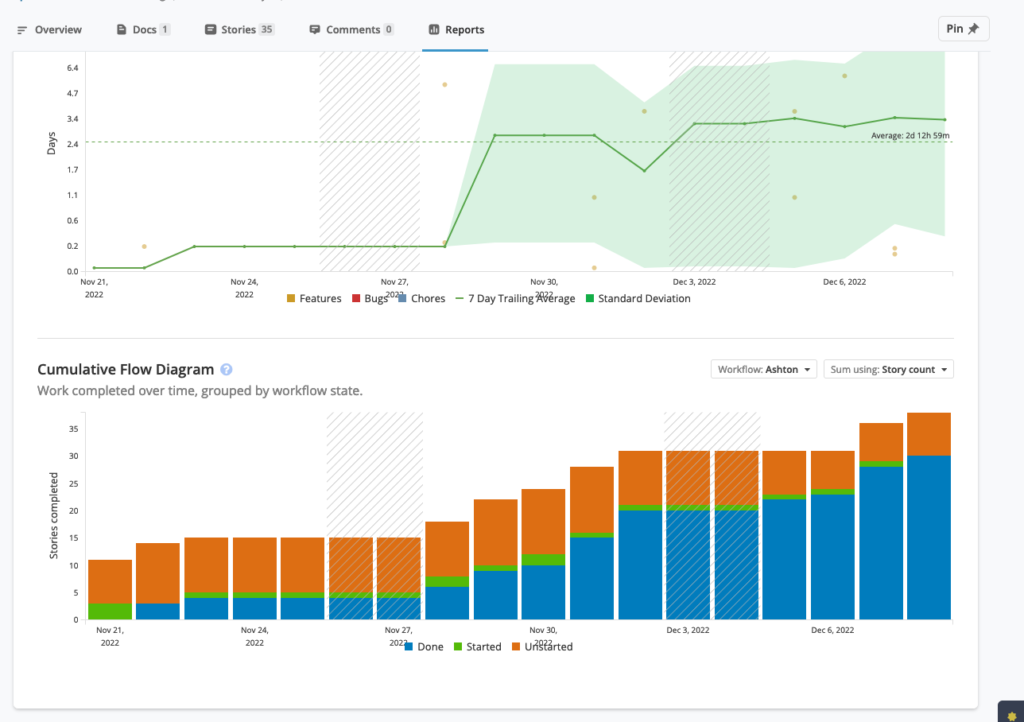
I used Shortcut.io to track open stories and work them down over time, and later clean up the project’s epic that I created. I’ve used issue trackers before, so it was nice to get out of using a makeshift kanban in a Google Doc table and go back to having a proper tracker for project management. A feature of project management that I love is being able to relate stories to each other in terms of which stories block or relate to other stories, because it helps me see which stories I need to prioritize.
Future Plans
After December 8, I closed out any remaining tasks and created new epics for Pet AI (for movement independent of the player) and randomly generating the Player’s Garden. Since the pet AI relies on being placed somewhere in an environment, and making pathing decisions within an environment, a blocking relationship between the two epics arises and when I resume work on this project it will be easier to see which tasks I need to complete first.
Demo
Conclusion
I have a ton of respect for the amount of effort that goes into creating sustained online game experiences, and a lot of ambition to join the ranks. I learned a ton getting this far and I am looking forward to learning more as I get this project restarted while taking on freelance work to pay my bills in the meantime.
Thank you for reading. Please let me know if there are any clarifications I can make or further questions I can answer either down in the comments, on LinkedIn, or hit me up on Mastodon.